Pushing Spring Boot Docker Image to AWS ECR & Setting Up GitLab CI/CD Pipelines
Key Topics covered:
- Pushing Spring Boot Docker Image to AWS ECR
- Setting up a .gitlab-ci.yml file to define your CI/CD pipeline.
- Creating an AWS IAM User
- Generating Access Keys
- Configuring GitLab CI/CD with Access Keys
- Pushing Docker images to the AWS ECR repository for deployment.
- Testing the CI/CD Pipeline
Description:
In this tutorial, we'll walk you through the step-by-step process of pushing a Spring Boot Docker image to Amazon Web Services (AWS) Elastic Container Registry (ECR). We'll also dive into creating a .gitlab-ci.yml configuration for GitLab CI/CD pipelines to automate image deployment. Additionally, we'll cover the creation of a user in AWS IAM and generating access keys specifically for pushing Docker images to your ECR repository.
1. Create AWS IAM User:
- Search for service IAM (Identity and Access Management)
- On Access Management, got to Users section
- Click button Create User
- Enter user name e.g ecr-ci-cd
- Click Next
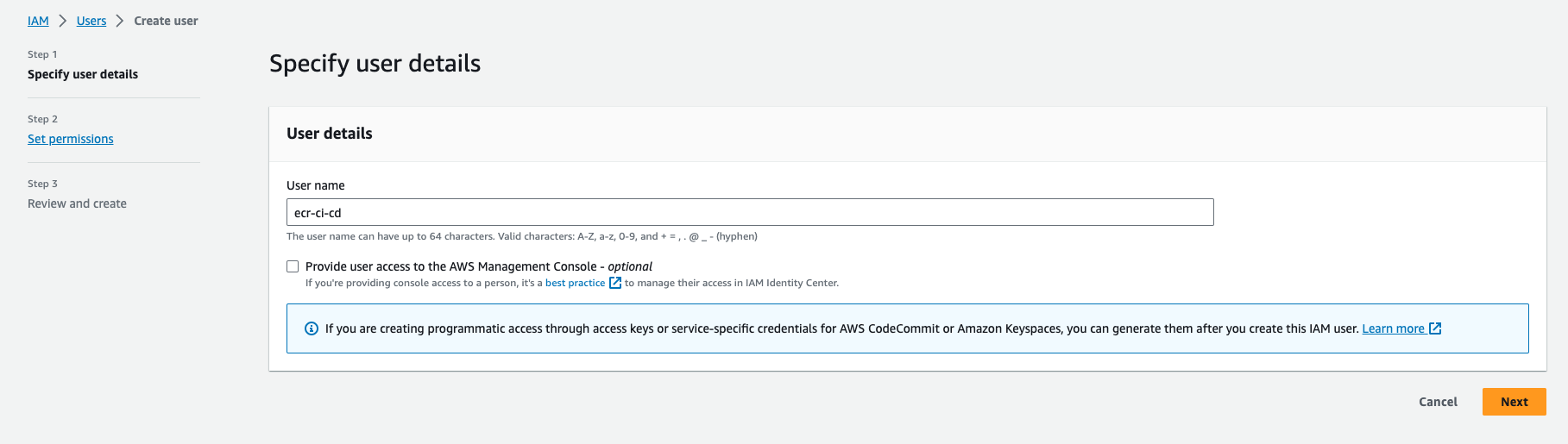
Create ECR User
1.1. Set User Permissions:
- On permissions options, choose Attach policies directly
- Search policy AmazonEC2ContainerRegistryFullAccess on Permissions polices and tick it
- Click Next
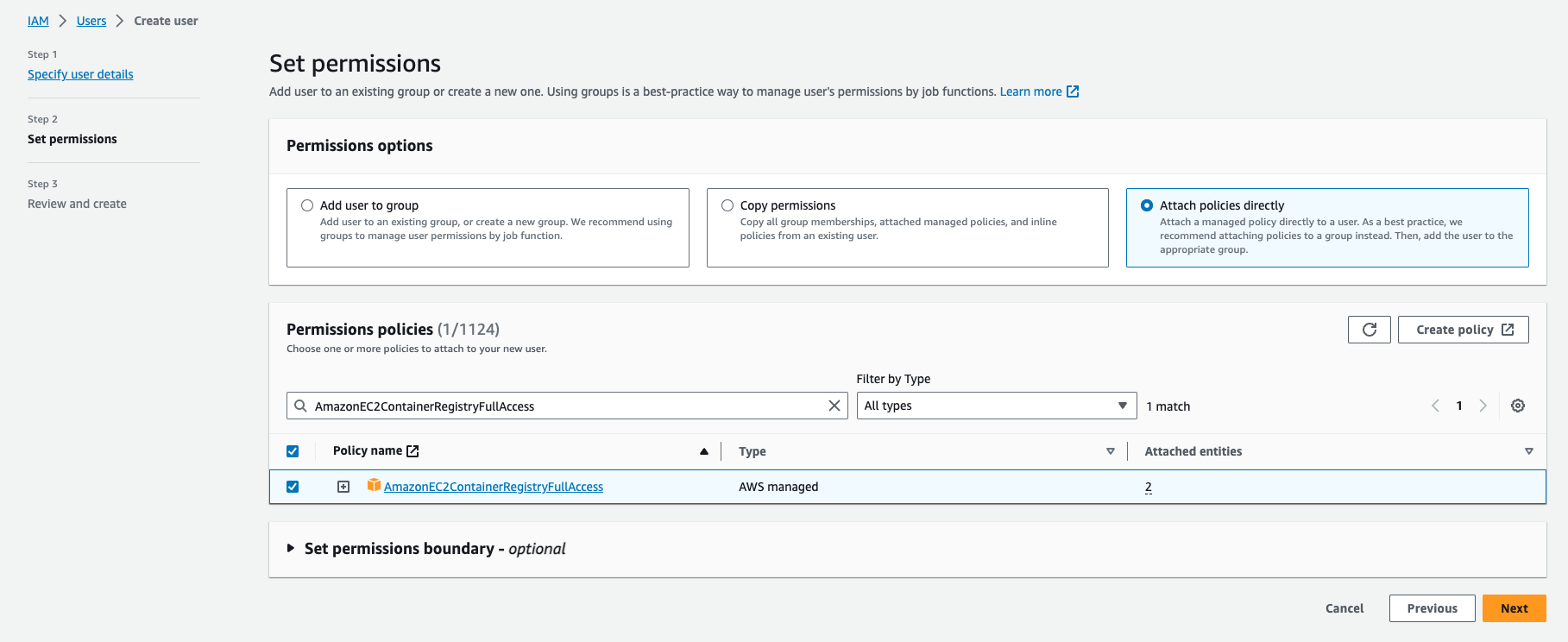
Set ECR User Permissions
1.2. Review and Create:
- Add Tags name to ECR CI/CD Push
- Click Create User Button
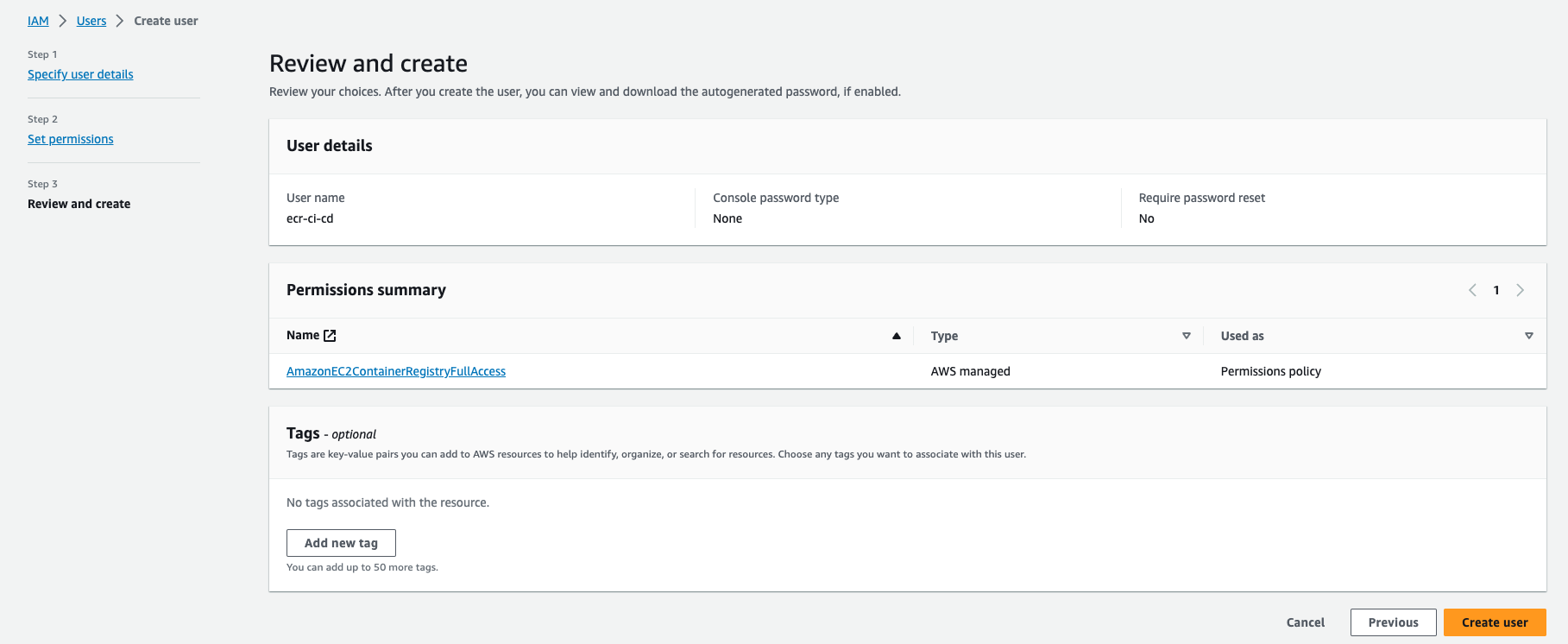
Review and Create
2. Create Access Tokens
Create your access token. Select the user ecr-ci-cd on list of users. Go to Security credential Tab. On Access Keys section, Click a button Create access key. Choose other and Click Next
Keep your access token somewhere safe and don't share it with anyone. We will need it when configuring our gilab-ci.
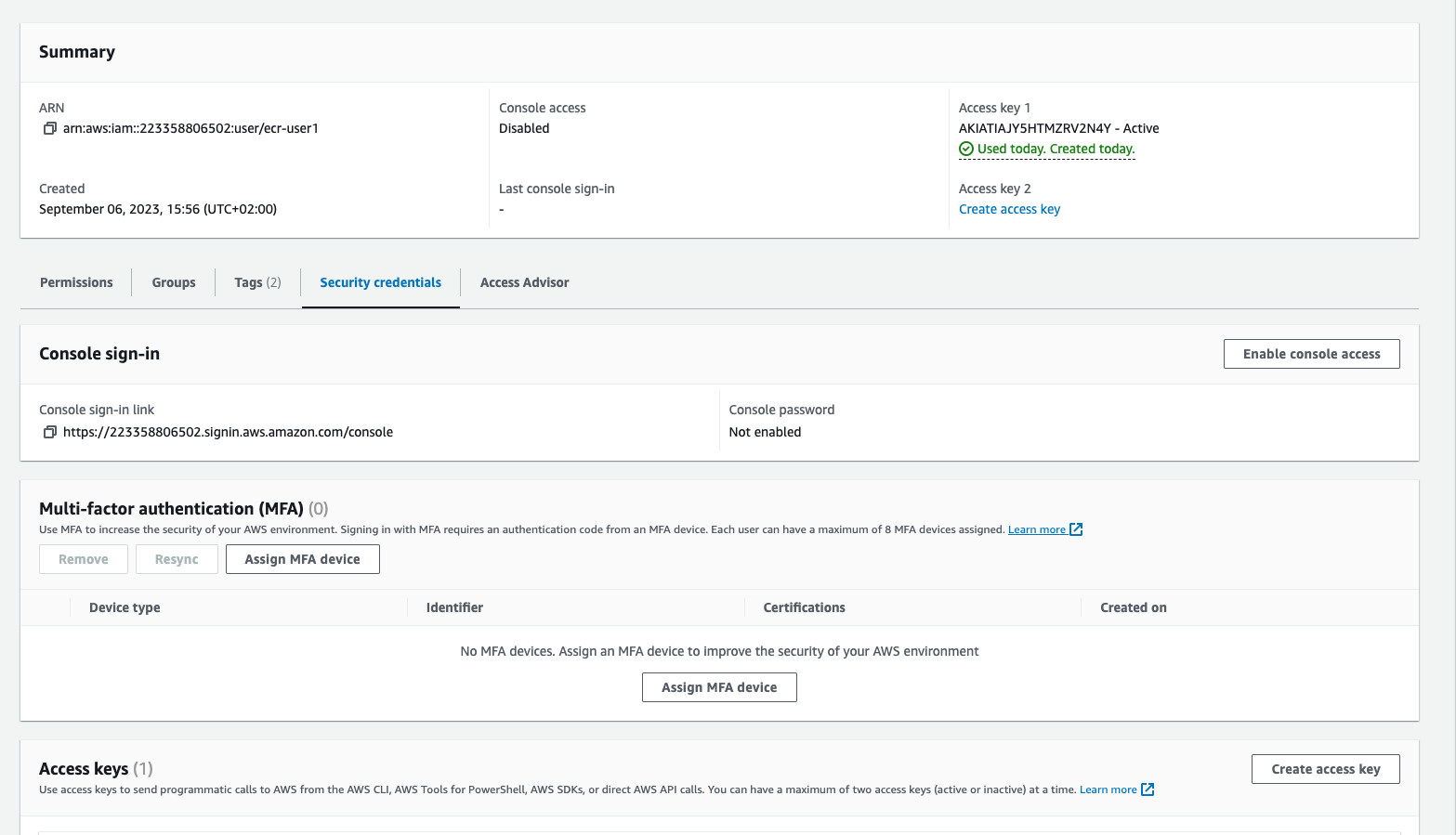
Choose other and Click Next
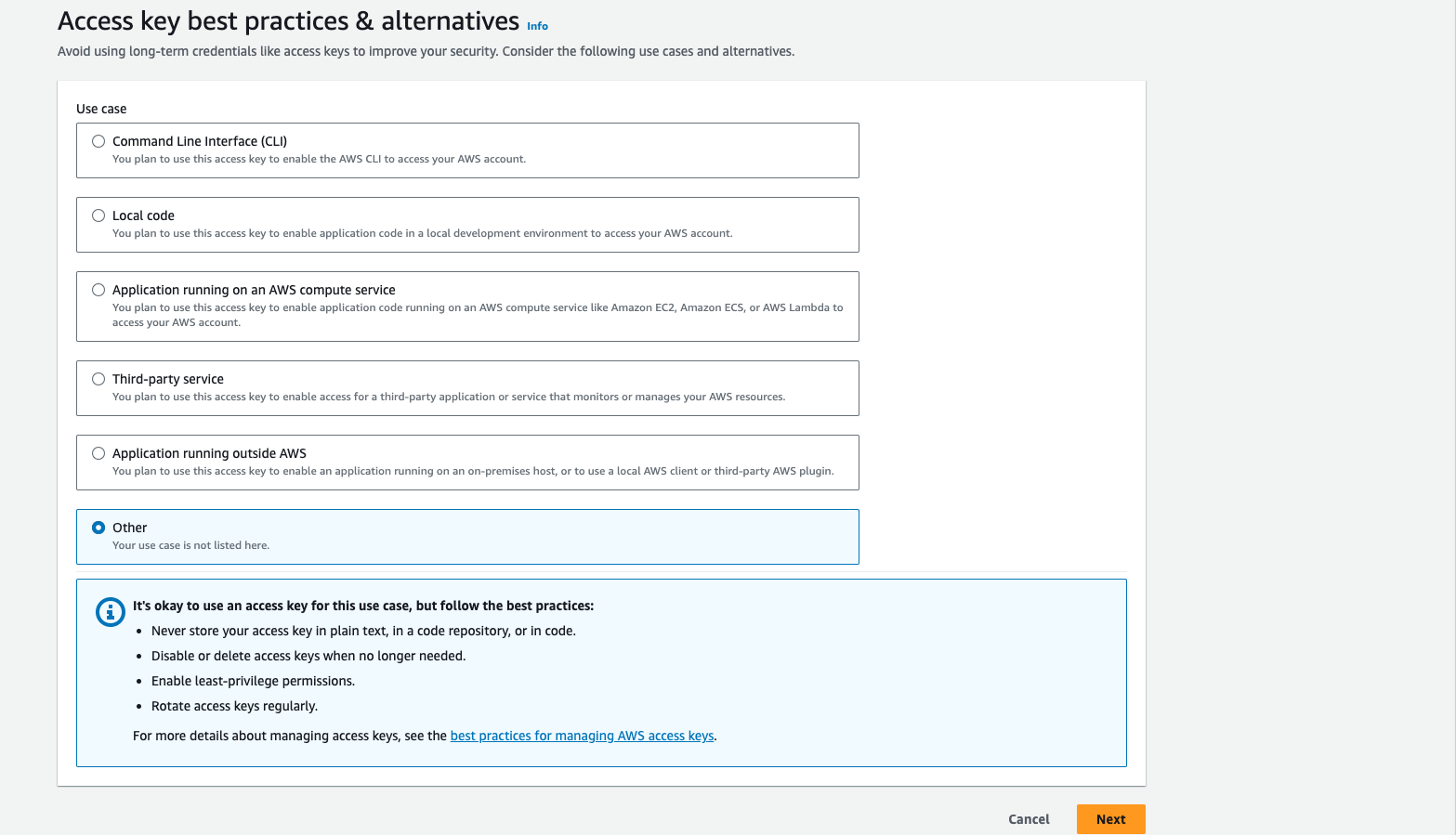
Click button Create access key
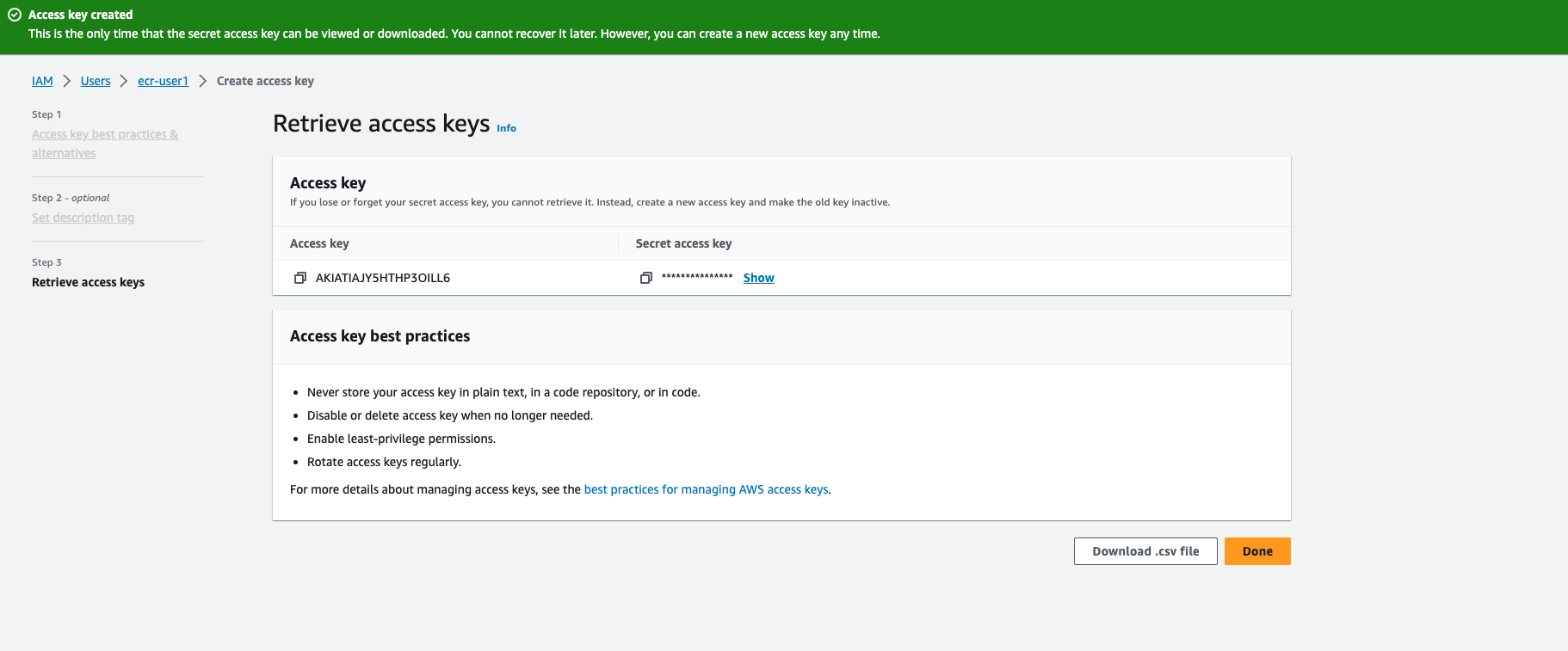
Click button Download .csv file Make sure you save this key and you won't retrieve them
3. Step-by-step guide to create a .gitlab-ci.yml:
Let's create a .gitlab-ci.yml in the root directory of the project
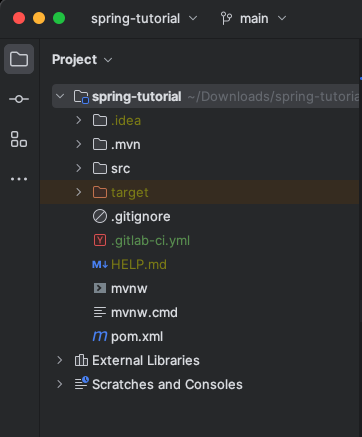
.gitlab-ci.yml in project root directory
100% working Springboot .gitlab-ci.yml
services:
- docker:19.03.7-dind
stages:
- build jar
- build and push docker image
- deploy
variables:
APPLICATION_NAME: "spring-boot-code-with-bisky-integrations"
TAG_NAME: "dev"
DOCKER_IMAGE: "codewithbisky/spring-boot-code-with-bisky-integrations"
build:
image: maven:3.8.3-openjdk-17
stage: build jar
script:
- mvn clean package
artifacts:
paths:
- target/*.jar
docker build:
image: docker:20.10.21-alpine3.17
stage: build and push docker image
rules:
- if: ($CI_COMMIT_BRANCH == "main" || $CI_COMMIT_REF_NAME == "main")
variables:
TAG_NAME: "latest"
- if: ($CI_COMMIT_BRANCH == "develop" || $CI_COMMIT_REF_NAME == "develop")
variables:
TAG_NAME: "develop"
script:
- apk add --update --no-cache curl py-pip
- pip install awscli
- aws configure set aws_access_key_id $AWS_ACCESS_KEY_ID
- aws configure set aws_secret_access_key $AWS_SECRET_ACCESS_KEY
- aws configure set region $AWS_REGION
- docker build -t $DOCKER_IMAGE .
- docker tag $DOCKER_IMAGE:latest 223358806502.dkr.ecr.eu-west-3.amazonaws.com/$DOCKER_IMAGE:$TAG_NAME
- aws ecr get-login-password --region eu-west-3 | docker login --username AWS --password-stdin 223358806502.dkr.ecr.eu-west-3.amazonaws.com
- docker push 223358806502.dkr.ecr.eu-west-3.amazonaws.com/$DOCKER_IMAGE:$TAG_NAME
Let me go through with you the instructions and commands above
services
The services section specifies the Docker image that will be used as a service during the CI/CD process. In this case, it uses the Docker image docker:19.03.7-dind, which includes Docker-in-Docker (DinD). This allows your CI/CD pipeline to run Docker commands inside the container, enabling you to build and push Docker images from within your CI/CD jobs
stages
The stages section defines the different stages of your CI/CD pipeline. Each stage represents a logical step in the process. The stages listed here are: "build jar," "build and push docker image," and "deploy." Jobs defined in the .gitlab-ci.yml file will be executed in the order of these stages
variables
The variables section defines environment variables that will be used throughout the pipeline. Here, you have defined the following variables:
- APPLICATION_NAME - The name of your Spring Boot application.
- TAG_NAME - The Docker image tag. It is initially set to "dev."
- DOCKER_IMAGE - The name of the Docker image to be built and pushed
build:
- This section defines the "build jar" job. It uses the maven:3.8.3-openjdk-17 Docker image, which contains the required Maven and Java tools.
- The job's script cleans and packages the Spring Boot application using Maven.
- he resulting JAR files are marked as artifacts to be passed to subsequent jobs or stored for future reference.
docker build:
- This section defines the "build and push docker image" job
- The job specifies different Docker image tags based on conditions using the rules keyword. For the "main" branch, it uses the "latest" tag, and for the "develop" branch, it uses the "develop" tag.
- The script installs AWS CLI, configures AWS access credentials and region, and then proceeds to build a Docker image from the JAR file.
- It tags the Docker image and pushes it to an Amazon ECR repository.
- Finally, it logs in to the ECR repository using AWS credentials and pushes the Docker image.
This GitLab CI/CD configuration automates the process of building a Spring Boot application, creating a Docker image, and pushing it to Amazon ECR. The pipeline is designed to handle different branches by assigning appropriate Docker image tags, allowing for version management of the deployed Docker images. Deployment to a specific environment (e.g., AWS ECS or Kubernetes) is typically done in the "deploy" stage, which is not detailed in this configuration but can be added as needed
3. Add Gitlab Project Variables
Add CI/CD Variables on your project settings
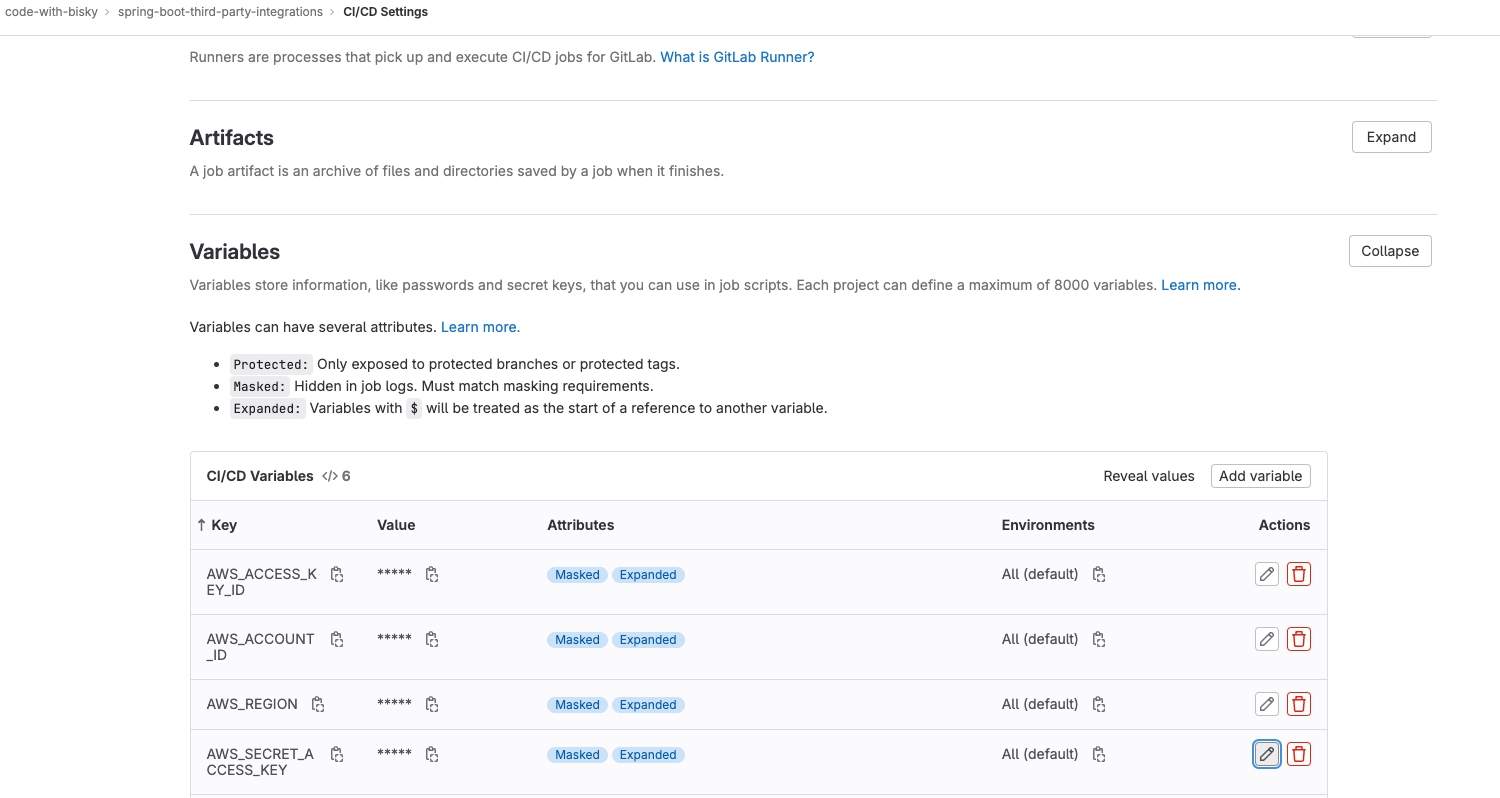
Add AWS_ACCESS_KEY_ID , AWS_ACCOUNT_ID, AWS_REGION , AWS_SECRET_ACCESS_KEY as variables.
- AWS_ACCESS_KEY_ID - Is the access key, take it from the csv file you downloaded
- AWS_SECRET_ACCESS_KEY - Is the secret access key, take it from the csv file you downloaded
- AWS_REGION - The region you created your ECR
- AWS_ACCOUNT_ID - Get it from your profile
Images showing successful CI/CD of our project
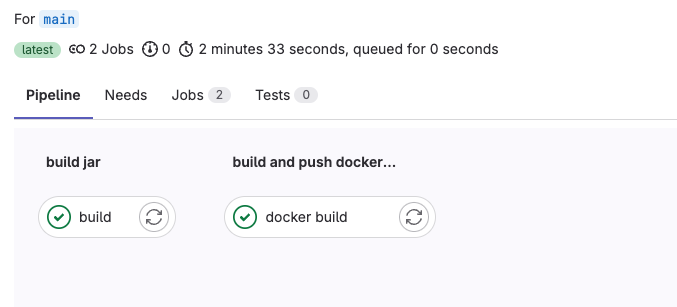
Success Pipeline
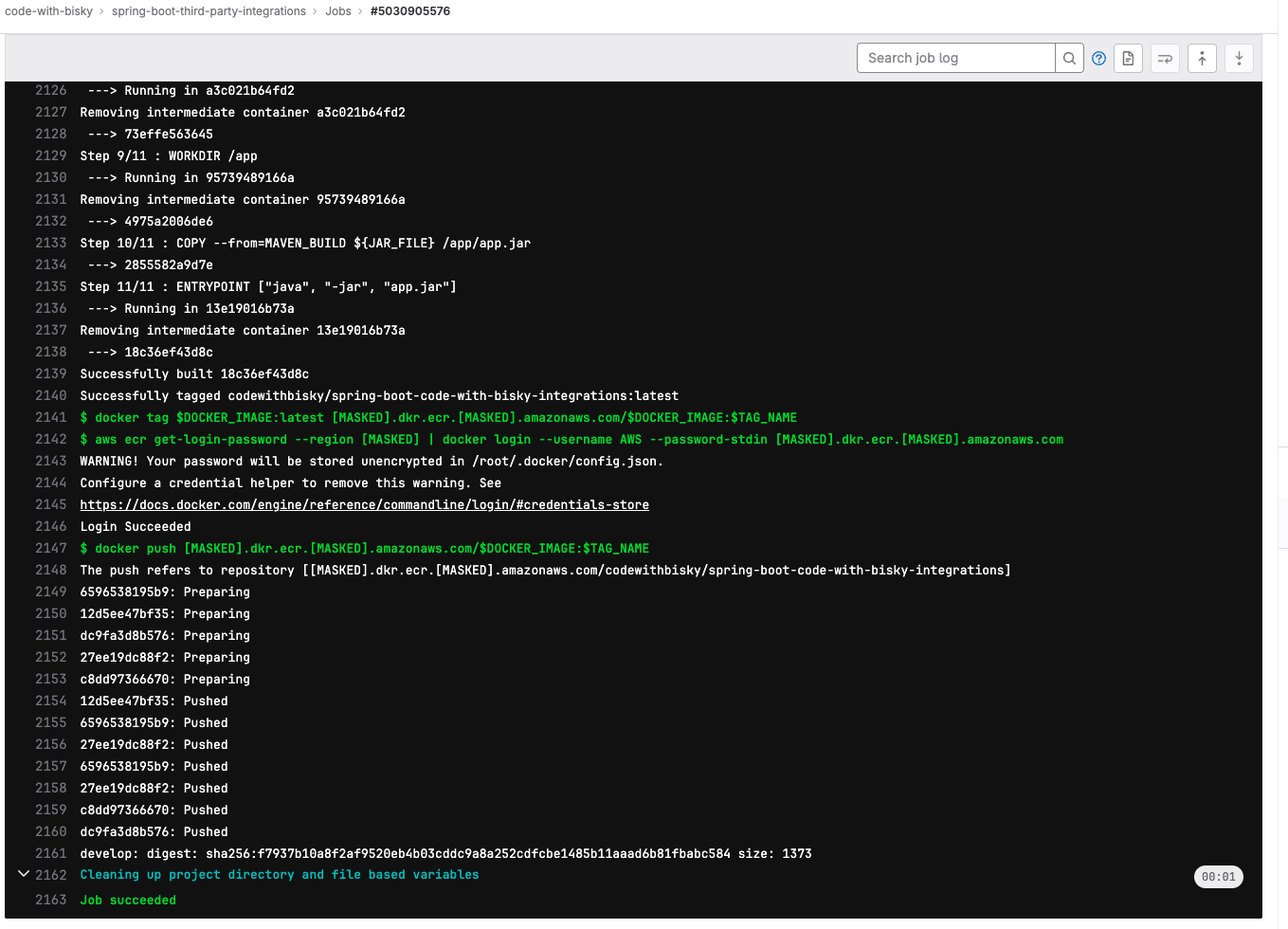
Push docker image to AWS ECR Pipeline
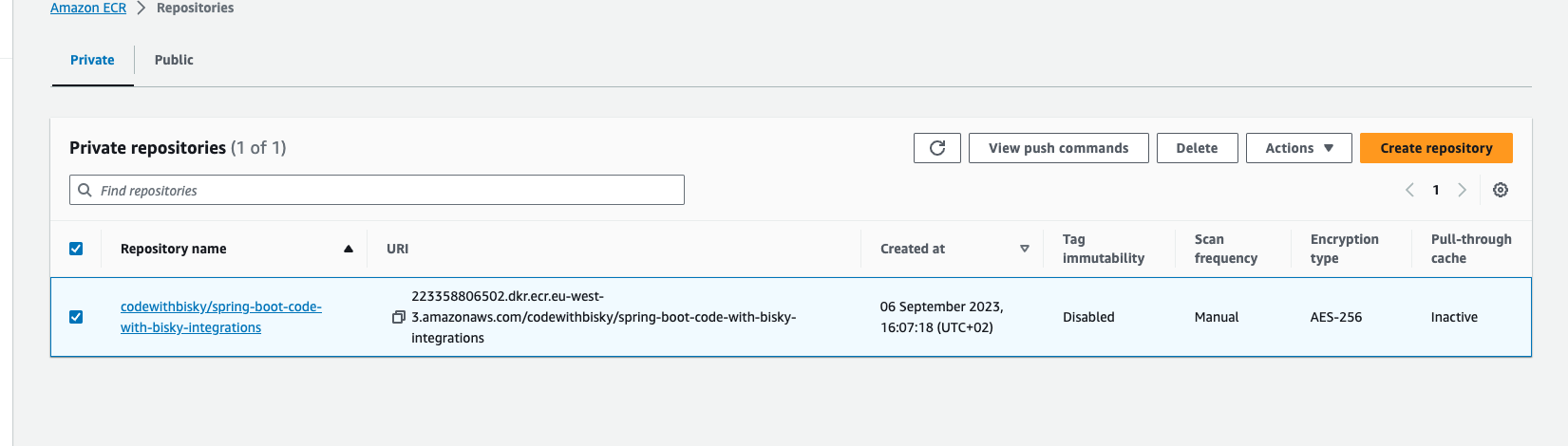
Pushed docker image to AWS ECR
Conclusion:
In this comprehensive tutorial, we've covered the essential steps for seamlessly integrating your Spring Boot applications with Amazon Web Services (AWS) Elastic Container Registry (ECR) and setting up GitLab CI/CD pipelines for automated deployments. Here's a quick recap of what we've accomplished:
- Pushing Spring Boot Docker Image to AWS ECR: We learned how to build and push a Spring Boot Docker image to AWS ECR, ensuring that your application is ready for deployment in the AWS cloud environment.
- Setting Up .gitlab-ci.yml Pipelines: We explored the creation of a .gitlab-ci.yml configuration file to automate the deployment process using GitLab CI/CD. This ensures that your application is consistently deployed whenever changes are made to your repository.
- Creating an AWS IAM User: To enhance security, we discussed the importance of creating a dedicated IAM user with limited permissions for Docker image management within AWS ECR.
- Generating Access Keys: We generated AWS access keys for the IAM user, which will be used in the GitLab CI/CD pipeline to securely push the Docker image to AWS ECR.
- Configuring GitLab CI/CD with Access Keys: We demonstrated how to configure GitLab CI/CD to use the generated access keys, ensuring a secure and automated deployment process.
- Testing the CI/CD Pipeline: Finally, we verified the functionality of our GitLab CI/CD pipeline, ensuring that our Spring Boot application is deployed automatically and correctly to AWS ECR.
By following these steps, you now have the knowledge and tools to streamline your Spring Boot application deployments to AWS ECR while maintaining security and automation through GitLab CI/CD. This tutorial empowers you to enhance your DevOps practices and accelerate your development workflow. Stay tuned for more insightful tutorials, and remember to like, subscribe, and hit the notification bell for future updates!